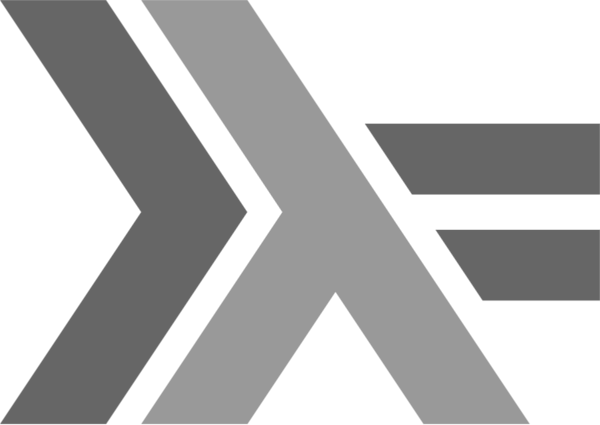
C++ is known for its many features (some argue there are too many!). Some significant programming paradigms supported by C++ are procedural, imperative, and object-oriented.
However, your friend, who is a programming language theorist, believes C++ is not very good because it isn't pure functional. Consider the following code:
int y = 0;
int f(int x) {
y = y+1;
return x*x;
}
int g(int z) {
return z+y;
}
We cannot substitute occurrences of g(f(5))
with g(25)
, since f
manipulates global state (side effects). Because of this, code is harder to reason about.
You'd like to challenge this belief. You decide to code a pure functional program in C++ that will solve the longest increasing subsequence problem.
However, in order to show that C++ can be coded in a pure functional way, you may not use #
, =
, or anything that mutates.
For full points, do not use *
, and try to use less than 2 MB of memory.
Input Specification
There is no input. Instead, there will be variables and functions already defined for you. In particular, we define:
const int N
N
, a variable representing the length of the sequence of integers.
constexpr int get_item(int i)
, a function that will return thei
element of the sequence
i
. All integers
in the sequence will satisfy
. Setting
i
out of range results in undefined behaviour.void print_num(int n)
, a function that will printn
tostdout
, followed by a newline (Technically, this function isn't pure functional, but we'll let it slide - after all, understanding pure functional I/O in Haskell is a difficult task on its own).
For example, your code might look like:
int main() {
print_num(N);
print_num(get_item(2));
return 0;
}
Output Specification
On the first line, output the length of the longest strictly increasing subsequence.
Then, on separate lines, output a longest strictly increasing subsequence. If there is more than one answer, output any of them.
Sample
Suppose N
and the sequence is
. Then one possible output is:
5
2
4
5
7
8
Notice
The spirit of the problem is to find a way to solve the problem without mutation, rather than trying to hack a method to bypass the mutation restriction. If your friend detects that your solution tries to bypass mutation, it will be rejected. If you repeatedly try to bypass mutation, your friend will assume you give up and will permanently ban you from submitting to the problem.
Comments
How does the scoring for this problem work? What is the penalty for using
*
and/or going over the 2MB memory limit?Edit: It seems like using * and less than 2MB is 30/100 and using no * but more than 2MB is 85/100.