The Great Sphinx has a riddle for you.
You are given a graph on
A sequence of vertices
There are
A path
You know the vertices and edges, but you do not know which colour each vertex has. You want to find out the colours of the vertices, by performing recolouring experiments.
In a recolouring experiment,
you may recolour arbitrarily many vertices.
Specifically, to perform a recolouring experiment
you first choose an array
, that is, the original colour of , if , or , otherwise.
Note that this means that you can use the Sphinx's colour in your recolouring.
Finally, the Great Sphinx announces
the number of monochromatic components in the graph,
after setting the colour of each vertex
Your task is to identify the colours of the vertices in the graph
by performing at most
Implementation Details
You should implement the following procedure.
std::vector<int> find_colours(int N,
std::vector<int> X, std::vector<int> Y)
: the number of vertices in the graph. , : arrays of length describing the edges.- This procedure should return an array
of length , representing the colours of vertices in the graph. - This procedure is called exactly once for each test case.
The above procedure can make calls to the following procedure to perform recolouring experiments:
int perform_experiment(std::vector<int> E)
: an array of length specifying how vertices should be recoloured.- This procedure returns the number of monochromatic components
after recolouring the vertices according to
. - This procedure can be called at most
times.
The grader is not adaptive, that is,
the colours of the vertices are fixed before a call to find_colours
is made.
Constraints
for each such that . or for each and such that .- Each pair of vertices is connected by some path.
for each such that .
Subtasks
Subtask | Score | Additional Constraints |
---|---|---|
1 | ||
2 | ||
3 | The graph is a path: |
|
4 | The graph is complete: |
|
5 | No additional constraints. |
In each subtask, you can obtain a partial score if your program determines correctly for every pair of adjacent vertices whether they have the same colour.
More precisely,
you get the whole score of a subtask
if in all of its test cases,
the array find_colours
is exactly the same as array
for each such that ;- For each
such that : if and only if .
Example
Consider the following call.
find_colours(4, [0, 1, 0, 0], [1, 2, 2, 3])
For this example, suppose that
the (hidden) colours of the vertices are given by
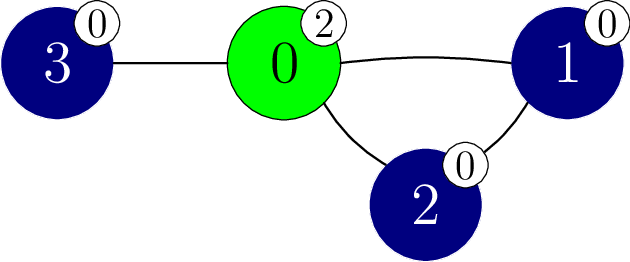
The procedure may call perform_experiment
as follows.
perform_experiment([-1, -1, -1, -1])
In this call, no vertex is recoloured, as all vertices keep their original colours.
Consider vertex
Consider vertex
Overall, there are
Now the procedure may call perform_experiment
as follows.
perform_experiment([0, -1, -1, -1])
In this call, only vertex
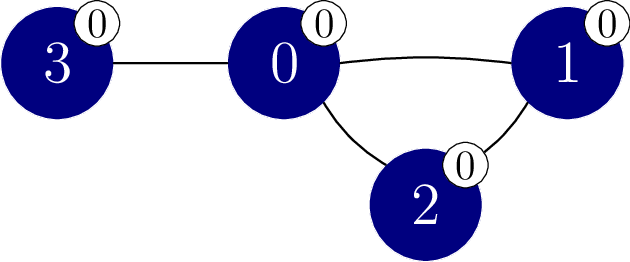
This call returns
The procedure may then call perform_experiment
as follows.
perform_experiment([-1, -1, -1, 2])
In this call, vertex
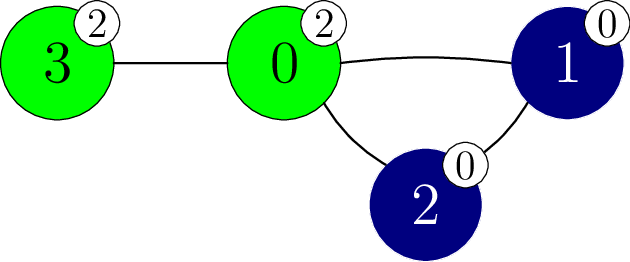
This call returns
The procedure find_colours
then returns the array
Note that there are also multiple return values, for which
Sample Grader
Input format:
N M
C[0] C[1] ... C[N-1]
X[0] Y[0]
X[1] Y[1]
...
X[M-1] Y[M-1]
Output format:
L Q
G[0] G[1] ... G[L-1]
Here, find_colours
,
and perform_experiment
.
Attachment Package
The sample grader along with sample test cases are available here.
Comments