In a nearby park, there are fountains, labeled from
to
. We model the fountains as points on a two-dimensional plane. Namely, fountain
is a point
where
and
are even integers. The locations of the fountains are all distinct.
Timothy the architect has been hired to plan the construction of some roads and the placement of one bench per road. A road is a horizontal or vertical line segment of length , whose endpoints are two distinct fountains. The roads should be constructed such that one can travel between any two fountains by moving along roads. Initially, there are no roads in the park.
For each road, exactly one bench needs to be placed in the park and assigned to (i.e., face) that road. Each bench must be placed at some point such that
and
are odd integers. The locations of the benches must be all distinct. A bench at
can only be assigned to a road if both of the road's endpoints are among
,
,
and
. For example, the bench at
can only be assigned to a road, which is one of the four line segments
,
,
,
.
Help Timothy determine if it is possible to construct roads, and place and assign benches satisfying all conditions given above, and if so, provide him with a feasible solution. If there are multiple feasible solutions that satisfy all conditions, you can report any of them.
Implementation Details
You should implement the following procedures:
int construct_roads(std::vector<int> x, std::vector<int> y)
,
: two arrays of length
. For each
, fountain
is a point
, where
and
are even integers.
- If a construction is possible, this procedure should make exactly one call to
build
(see below) to report a solution, following which it should return.
- Otherwise, the procedure should return
without making any calls to
build
. - This procedure is called exactly once.
Your implementation can call the following procedure to provide a feasible construction of roads and a placement of benches:
void build(std::vector<int> u, std::vector<int> v, std::vector<int> a, std::vector<int> b)
- Let
be the total number of roads in the construction.
,
: two arrays of length
, representing the roads to be constructed. These roads are labeled from
to
. For each
, road
connects fountains
and
. Each road must be a horizontal or vertical line segment of length
. Any two distinct roads can have at most one point in common (a fountain). Once the roads are constructed, it should be possible to travel between any two fountains by moving along roads.
,
: two arrays of length
, representing the benches. For each
, a bench is placed at
, and is assigned to road
. No two distinct benches can have the same location.
Examples
Example 1
Consider the following call:
construct_roads({4, 4, 6, 4, 2}, {4, 6, 4, 2, 4})
This means that there are fountains:
- fountain
is located at
,
- fountain
is located at
,
- fountain
is located at
,
- fountain
is located at
,
- fountain
is located at
.
It is possible to construct the following roads, where each road connects two fountains, and place the corresponding benches:
Road label | Labels of the fountains the road connects | Location of the assigned bench |
---|---|---|
This solution corresponds to the following diagram:
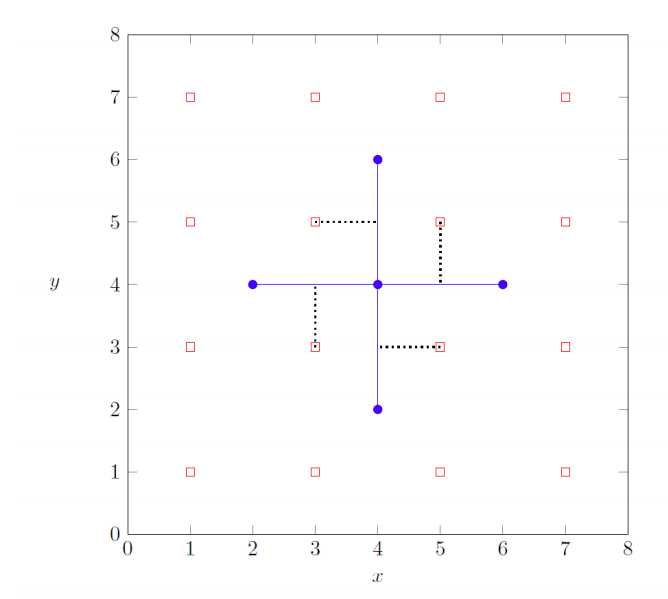
To report this solution, construct_roads
should make the following call:
build({0, 0, 3, 4}, {2, 1, 0, 0}, {5, 3, 5, 3}, {5, 5, 3, 3})
It should then return .
Note that in this case, there are multiple solutions that satisfy the requirements, all of which would be considered correct. For example, it is also correct to call build({1, 2, 3, 4}, {0, 0, 0, 0}, {5, 5, 3, 3}, {5, 3, 3, 5})
and then return .
Example 2
Consider the following call:
construct_roads({2, 4}, {2, 6})
Fountain is located at
and fountain
is located at
. Since there is no way to construct roads that satisfy the requirements,
construct_roads
should return without making any call to
build
.
Constraints
(for all
)
and
are even integers (for all
)
- No two fountains have the same location.
Subtasks
- (
points)
(for all
)
- (
points)
(for all
)
- (
points)
(for all
)
- (
points) There is at most one way of constructing the roads, such that one can travel between any two fountains by moving along roads.
- (
points) There do not exist four fountains that form the corners of a
square.
- (
points) No additional constraints.
Sample Grader
The sample grader reads the input in the following format:
- line
:
- line
:
The output of the sample grader is in the following format:
- line
: the return value of
construct_roads
If the return value of construct_roads
is and
build(u, v, a, b)
is called, the grader then additionally prints:
- line
:
- line
:
Attachment Package
The sample grader and sample test cases are available here: parks.zip.
Comments