Adnan owns the biggest shoe store in Baku. A box containing pairs of shoes has just
arrived at the store. Each pair consists of two shoes of the same size: a left and a right
one. Adnan has put all of the
shoes in a row consisting of
positions numbered
through
from left to right.
Adnan wants to rearrange the shoes into a valid arrangement. An arrangement is
valid if and only if for every
, the following conditions hold:
- The shoes at positions
and
are of the same size.
- The shoe at position
is a left shoe.
- The shoe at position
is a right shoe.
For this purpose, Adnan can make a series of swaps. In each swap, he selects two shoes that are adjacent at that moment and exchanges them (i.e., picks them up and puts each one on the former position of the other shoe). Two shoes are adjacent if their positions differ by one.
Determine the minimum number of swaps that Adnan needs to perform in order to obtain a valid arrangement of the shoes.
Implementation details
You should implement the following procedure:
long long count_swaps(std::vector<int> S)
: an array of
integers. For each
,
is a non-zero value equal to the size of the shoe initially placed at position
. Here,
denotes the absolute value of
, which equals
if
and equals
if
. If
, the shoe at position
is a left shoe; otherwise, it is a right shoe.
- This procedure should return the minimum number of swaps (of adjacent shoes) that need to be performed in order to obtain a valid arrangement.
Examples
Example 1
Consider the following call:
count_swaps({2, 1, -1, -2})
Adnan can obtain a valid arrangement in swaps.
For instance, he can first swap shoes and
, then
and
, then
and
, and
finally
and
. He would then obtain the following valid arrangement:
.
It is not possible to obtain any valid arrangement with less than
swaps. Therefore,
the procedure should return
.
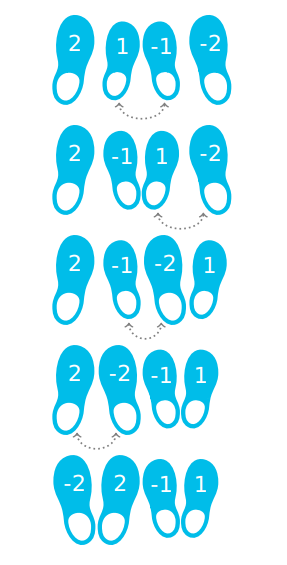
Example 2
In the following example, all the shoes have the same size:
count_swaps({-2, 2, 2, -2, -2, 2})
Adnan can swap the shoes at positions and
to obtain the valid arrangement
, so the procedure should return
.
Constraints
- For each
,
.
- A valid arrangement of the shoes can be obtained by performing some sequence of swaps.
Subtasks
- (
points)
- (
points)
- (
points) All the shoes are of the same size.
- (
points) All shoes at positions
are left shoes, and all shoes at positions
are right shoes. Also, for each
, the shoes at positions
and
are of the same size.
- (
points)
- (
points) No additional constraints.
Sample grader
The sample grader reads the input in the following format:
- line
:
- line
:
The sample grader outputs a single line containing the return value of count_swaps
.
Comments
Why is there only one language allowed?
DMOJ only supports signature grading in C/++. As the IOI no longer supports C, this leaves C++ as the only language supported by this problem.
Note that as of 2021, IOI also no longer supports Java.
Can anyone explain the BIT solution
Hint: check what should you update whenever you move the chosen shoe and try updating that with a BIT.
Hello! I coded some subtask and DMOJ gave me WA. Looking at the test cases I found my program works fine, but there are some strange codes in the first line of each case. Could you check that, please? :-)
It's a very basic anti-cheating measure employed by the IOI. Unless you're trying to bypass the signature grader it shouldn't matter.