The city of Tehran is home to the National Library of Iran. The main treasure of this library is located in a long hall with a row of
Aryan, a librarian, is going to do the job. He has created a list
Aryan starts sorting the books at table
- If he is not carrying a book and there is a book on the table he is at, he can pick up this book.
- If he is carrying a book and there is another book on the table he is at, he can switch the book he is carrying with the one on the table.
- If he is carrying a book and he is at an empty table, he can put the book he is carrying on the table.
- He can walk to any table. He may carry a single book while he does so.
For all
Implementation details
You should implement the following procedure:
long long minimum_walk(std::vector<int> p, int s)
is an array of length . The book that is on table at the beginning should be taken by Aryan to table (for all ). is the label of the table where Aryan is at the beginning, and where he should be after sorting the books.- This procedure should return the minimum total distance (in meters) Aryan has to walk in order to sort the books.
Example
minimum_walk({0, 2, 3, 1}, 0)
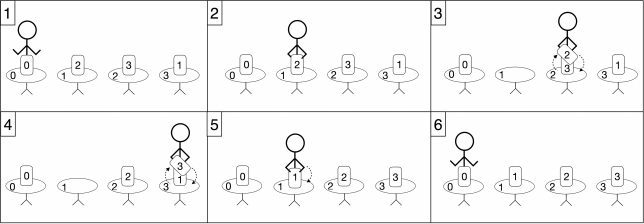
In this example,
- He walks to table
and picks up the book lying on it. This book should be put on table . - Then, he walks to table
and switches the book he is carrying with the book on the table. The new book he is carrying should be put on table . - Then, he walks to table
and switches the book he is carrying with the book on the table. The new book he is carrying should be put on table . - Then, he walks to table
and puts the book he is carrying on the table. - Finally, he walks back to table
.
Note that book on table
Constraints
- Array
contains distinct integers between and , inclusive.
Subtasks
- (12 points)
and - (10 points)
and - (28 points)
- (20 points)
- (30 points) no additional constraints
Sample grader
The sample grader reads the input in the following format:
- line
: - line
:
The sample grader prints a single line containing the return value of minimum_walk
.
Comments